In this article, we will create custom middleware. Middleware is a mechanism for filtering the HTTP request.
Here we will create a custom middleware for checking the request type is JSON or not. This middleware will filter the request and return the error response if the request type is not JSON. So let’s start.
Create Middleware:
First, we will open the terminal and run the below command.
php artisan make:middleware checkJsonRequest
Register Custom Middleware
The above command will create a middleware file. Now go to the file app/Http/kernal.php file and register the custom middleware.
namespace App\Http;
use Illuminate\Foundation\Http\Kernel as HttpKernel;
class Kernel extends HttpKernel
{
/**
* The application's route middleware.
*
* These middleware may be assigned to groups or used individually.
*
* @var array
*/
protected $routeMiddleware = [
'checkJsonRequest' => \App\Http\Middleware\checkJsonRequest::class,
];
}
Implement Logic in Custom Middleware
After registering custom middleware, we need to implement our logic into the custom middleware file. Go to the app/Http/Middleware and implement the logic:
Open custom middleware file: app/Http/Middleware/checkJsonRequest.php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
class checkJsonRequest
{
/**
* Handle an incoming request.
*
* @param \Illuminate\Http\Request $request
* @param \Closure $next
* @return mixed
*/
public function handle(Request $request, Closure $next)
{
if (empty($request->json()->all())) {
return response()->json([
'message' => 'The request is not a valid JSON.',
], 400);
}
return $next($request);
}
}
Create Route
Now we will create a simple route and use our custom middleware. Here we are creating this custom middleware to validate API JSON payload so open the api.php file. If you need this custom middleware for web routes then simply you can use this in web.php. It will work fine.
Path: routes/api.php
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\API\AuthController;
Route::post('user', [AuthController::class, 'user'])->middleware('checkJsonRequest');
Test Middleware
Now we need to check our middleware is working or not. Open postman and hit the URL.
Please look into the below image. If the request type is not JSON, API gives the error message.
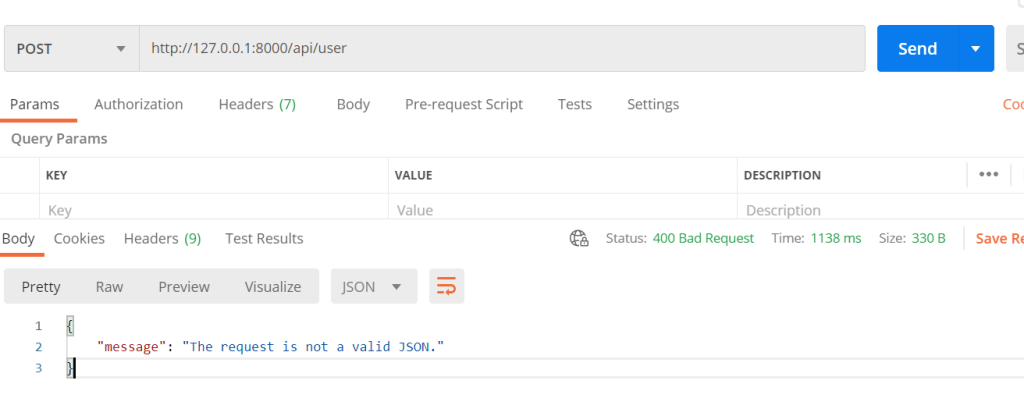
Thanks for reading, feel free to reach out to me for any comments and suggestions. I hope you found the article helpful and you were able to create custom middleware.