When you want to restrict the user to access a specific area, then we need to implement 2-way authentication. There are many packages available on the internet to create the OTP functionality and you can use that easily. In this article, I will show you how to create OTP functionality without any Laravel packages and how to log in with OTP. So let’s start.
Here I will create 2 APIs, the first API is for the request API, and the second is for the OTP verification.
Simple Steps to send the OTP
Step 1: Create Routes
First, create the routes for the API.
Route::any('request_otp', 'API\AuthController@requestOtp');
Route::post('verify_otp', 'API\AuthController@verifyOtp');
Step 2:
Add the OTP column to the users table. In this column, we will save and remove the generated OTP.
Step 3:
Now we need to create request OTP functionality. In the below code, I have generated the OTP and save the generated OTP in the users table’s OTP column. After that, I sent the OTP in the email. If you have any issues with email functionality. You can check my other article to set up email functionality in the laravel.
public function requestOtp(Request $request)
{
$otp = rand(1000,9999);
Log::info("otp = ".$otp);
$user = User::where('email','=',$request->email)->update(['otp' => $otp]);
if($user){
$mail_details = [
'subject' => 'Testing Application OTP',
'body' => 'Your OTP is : '. $otp
];
\Mail::to($request->email)->send(new sendEmail($mail_details));
return response(["status" => 200, "message" => "OTP sent successfully"]);
}
else{
return response(["status" => 401, 'message' => 'Invalid']);
}
}
Step 4:
After creating the request OTP API, now we will create OTP verification API. In the below code, I have checked the email and OTP is correct or not. If OTP is correct then create the login functionality and provide the access token in the response.
public function verifyOtp(Request $request){
$user = User::where([['email','=',$request->email],['otp','=',$request->otp]])->first();
if($user){
auth()->login($user, true);
User::where('email','=',$request->email)->update(['otp' => null]);
$accessToken = auth()->user()->createToken('authToken')->accessToken;
return response(["status" => 200, "message" => "Success", 'user' => auth()->user(), 'access_token' => $accessToken]);
}
else{
return response(["status" => 401, 'message' => 'Invalid']);
}
}
API Testing
I use Postman to test the API and for the API documentation. I sent the request params in the Form data. Please see the below screenshots.
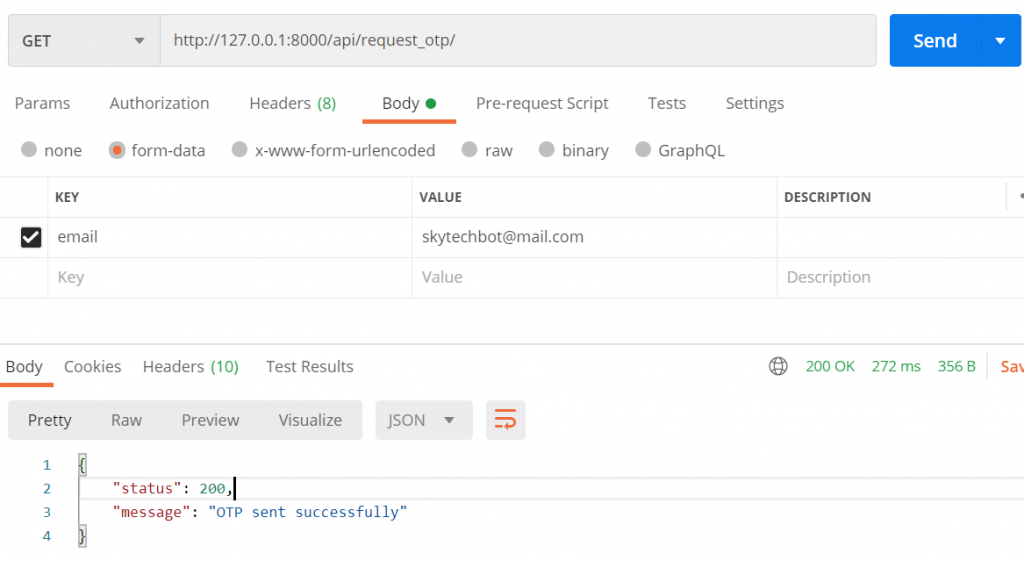
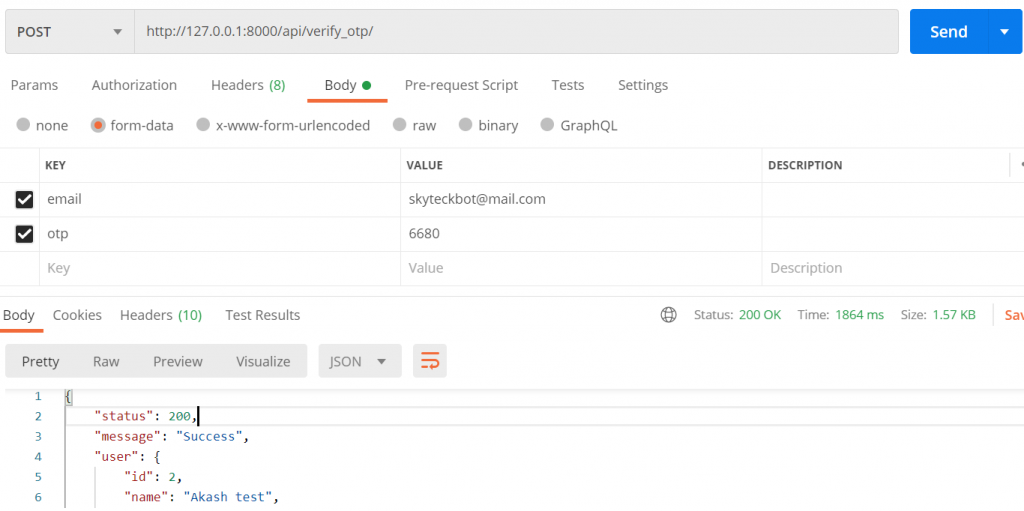
Now everything is done. This was a very simple approach to send the OTP in the email without any laravel package.
Full Controller code:
<?php
namespace App\Http\Controllers\API;
use App\Http\Controllers\Controller;
use App\User;
use Validator;
use Illuminate\Http\Request;
use Exception;
use Log;
use Illuminate\Support\Facades\Hash;
use Illuminate\Support\Facades\Password;
use Illuminate\Support\Facades\Mail;
use App\Mail\sendEmail;
class AuthController extends Controller
{
public function requestOtp(Request $request)
{
$otp = rand(1000,9999);
Log::info("otp = ".$otp);
$user = User::where('email','=',$request->email)->update(['otp' => $otp]);
if($user){
// send otp in the email
$mail_details = [
'subject' => 'Testing Application OTP',
'body' => 'Your OTP is : '. $otp
];
\Mail::to($request->email)->send(new sendEmail($mail_details));
return response(["status" => 200, "message" => "OTP sent successfully"]);
}
else{
return response(["status" => 401, 'message' => 'Invalid']);
}
}
public function verifyOtp(Request $request){
$user = User::where([['email','=',$request->email],['otp','=',$request->otp]])->first();
if($user){
auth()->login($user, true);
User::where('email','=',$request->email)->update(['otp' => null]);
$accessToken = auth()->user()->createToken('authToken')->accessToken;
return response(["status" => 200, "message" => "Success", 'user' => auth()->user(), 'access_token' => $accessToken]);
}
else{
return response(["status" => 401, 'message' => 'Invalid']);
}
}
}
Note:
You can also use the below packages to create OTP functionality. I will let you know about these packages in my next articles.
Some Best Laravel Packages for the OTP:
https://github.com/antonioribeiro/google2fa-laravel
https://github.com/aloha/laravel-twilio
https://github.com/ichtrojan/laravel-otp
https://github.com/seshac/otp-generator
https://github.com/ferdousulhaque/laravel-otp-validate
Thanks for reading, feel free to reach out to me for any comments and suggestions. I hope you found the article helpful and you were able to send OTP in the email